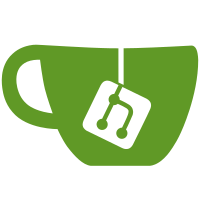
* Add the first test and upgrade build.gradle to modern standards
1. gradle wrapper
2. ./gradlew run
3. manifest will look like
Manifest-Version: 1.0
Main-Class: me.topchetoeu.jscript.runtime.SimpleRepl
Build-Timestamp: 2024-09-04T10:44:35.990+0200
Build-Branch: ma/add-first-tests
Build-Revision: 412edc0ebc
Build-Jdk: 21.0.3 (Oracle Corporation 21.0.3+7-LTS-152)
Build-Author: TopchetoEU
4. build/distributions contains a zip and a jar which contain jscript-0.9.41-beta.jar
5. unnecessary libs have been removed
6. gradle has been updated to 8.10
7. first test has been added
* fix: revert removal of Jabel (for support of Java 11)
---------
Co-authored-by: TopchetoEU <36534413+TopchetoEU@users.noreply.github.com>
35 lines
1.3 KiB
Java
35 lines
1.3 KiB
Java
package me.topchetoeu.jscript.lib;
|
|
|
|
import me.topchetoeu.jscript.runtime.Context;
|
|
import me.topchetoeu.jscript.runtime.Extensions;
|
|
import me.topchetoeu.jscript.runtime.Frame;
|
|
import me.topchetoeu.jscript.runtime.exceptions.EngineException;
|
|
import me.topchetoeu.jscript.runtime.values.CodeFunction;
|
|
import me.topchetoeu.jscript.runtime.values.FunctionValue;
|
|
import me.topchetoeu.jscript.runtime.values.NativeFunction;
|
|
import me.topchetoeu.jscript.utils.interop.WrapperName;
|
|
|
|
@WrapperName("AsyncGeneratorFunction")
|
|
public class AsyncGeneratorFunctionLib extends FunctionValue {
|
|
public final CodeFunction func;
|
|
|
|
@Override
|
|
public Object call(Extensions ext, Object thisArg, Object ...args) {
|
|
var handler = new AsyncGeneratorLib();
|
|
|
|
var newArgs = new Object[args.length + 2];
|
|
newArgs[0] = new NativeFunction("await", handler::await);
|
|
newArgs[1] = new NativeFunction("yield", handler::yield);
|
|
System.arraycopy(args, 0, newArgs, 2, args.length);
|
|
|
|
handler.frame = new Frame(Context.of(ext), thisArg, newArgs, func);
|
|
return handler;
|
|
}
|
|
|
|
public AsyncGeneratorFunctionLib(CodeFunction func) {
|
|
super(func.name, func.length);
|
|
if (!(func instanceof CodeFunction)) throw EngineException.ofType("Return value of argument must be a js function.");
|
|
this.func = func;
|
|
}
|
|
}
|